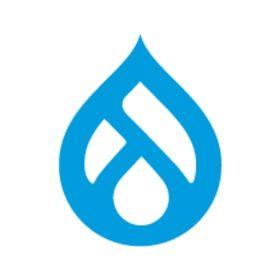
I'd like to display a certain block (the events calendar block in case it matters) only when users are in a certain book on my website (the 'book' that deals with our community activities, signing up, past event reports etc).
Is there a simple PHP snippet I can use to detect if the page being displayed is in the book whose root node ID is some value?
Or, perhaps a different approach.
Is there a simple PHP snippet I can use to detect which Primary Nav bar tab the page is in? (Each tab corresponds approximately to a different book). Then I could just have the Event module display when the user is in a certain tab.
thx, michael
Comments
Mmm...
It may be too late for this but...
When I create a book, I usually give all sections of the book the following url alias : book_name/section_name
So I can access section1 at book_name/section1_name
and have section2 at book_name/section2_name
and I can access the book 'cover' at book_name
Now...
For the block visibility, I would use php and...
I am sure there is another way though...
Caroline
A coder's guide to file download in Drupal
Who am I | Where are we
11 heavens
Database
A full-proof way is to look up the database.
I forgot to return false in my other snippet in the case where we are not on page with internal path
node/nid
.Caroline
A coder's guide to file download in Drupal
Who am I | Where are we
11 heavens
This code seems to work
Thanks Caroline. You got me started but the code didn't quite work. This does seem to work however. Note that the node ID of the book I'm checking against is 46, so others should substitute as need be:
Not sure how important the upper case TRUE/FALSE is, but the extra db_fetch_object was critical. Perhaps there's a better way as the result should be a singleton.
thanks again! michael
--
true or TRUE, it does not matter in php. My guess is that even when getting a single value (one column with one value in that column, no 'object' per say), we still have to use db_fetch_object.
I always use db_fetch_object in my code anyway, because I am not a Mysql-in-Drupal expert... That's what I put first up there, then edited myself. Actually my first trial was too wordy (using b without necessity) :
$result = db_fetch_object(db_query("SELECT b.parent FROM {book} b WHERE b.nid = %d", arg(1)));
That's first rate code you have there. :b <--- that's me drooling
Thanks for sharing!
Caroline
A coder's guide to file download in Drupal
Who am I | Where are we
11 heavens
Slightly different way without db_query
$node->parent is loaded by default me believes so you may use that instead of making a query. The Book nid will be 42 and all child pages will have $node->parent = 42
One Love
a better way
blocks don't load the node, hence the arg and nid approach. this will do what you want, but only works in Drupal 6.
parent method will work, but only two levels deep. you'll have to add a bit more logic if you want it to work with children of children.
Added to PHP Block Snippets
I've added this to the PHP tricks section of the docs: http://drupal.org/node/125380 - please update there if you think its appropriate. Thanks!
thank you
That's great :) I looked at the page. That code is top notch.
Caroline
Who am I | Where are we
11 heavens
would only work for the first two levels
this would only work for the top level and first level. Not if you do have more levels (like book title - chapter - paragraph. This would only show up at title and chapter, not at paragraph pages)
I have modified this script
I have modified this script to support more than 2 levels of book hierarchy.
Note, you need to replace 46 with your own book id (the most top level book node).
This code works on Drupal 6.
Any book
Hi
It's slightly off topic, but I just need to check if the page belongs to a book (no specific, any book ok).
So maybe we can work with
But unfortunately I can't code php.
So I would be happy for a suggestion.
Thanks for your help :)
If possible, I would like to
If possible, I would like to combine it with:
Thanks a lot for your help :)